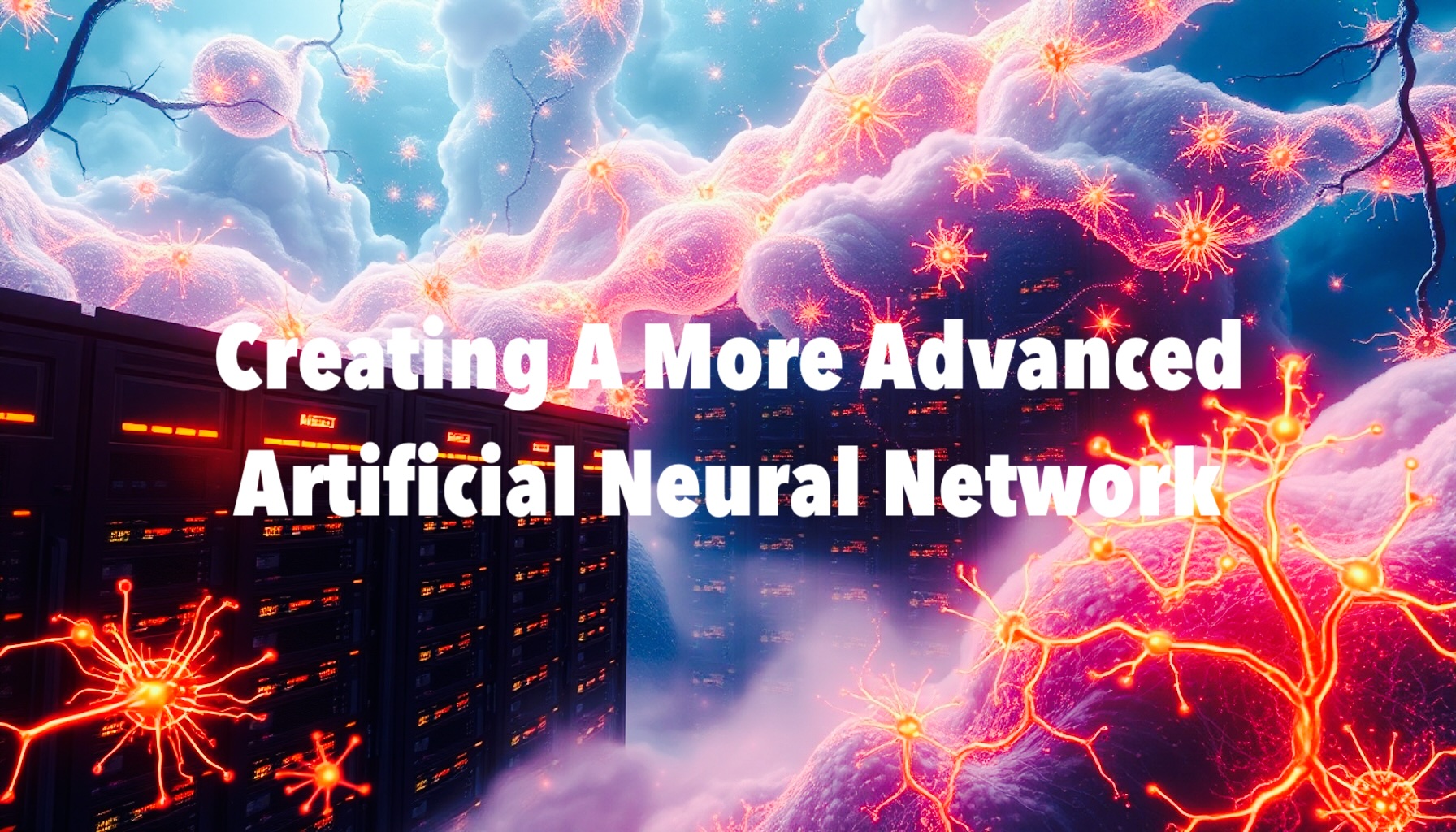
The basic artificial neural network we created doesn’t have the best accuracy. Though it performed somewhat well in the recognition of MNIST test data images, it didn’t do as well in the recognition of real-world images. To improve its performance, we can do some adjustments to our model and training data.
Continue reading “Creating A More Advanced Artificial Neural Network”