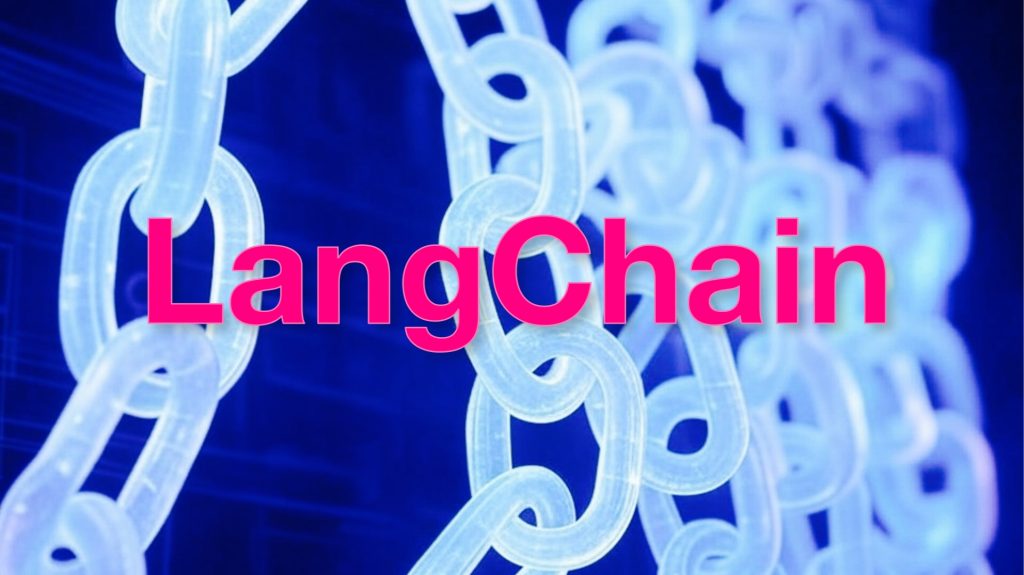
LangChain is an open-source framework designed to help developers build applications that leverage language models (LLMs). It provides tools and abstractions to simplify the development of applications centered around natural language processing (NLP), such as language generation, retrieval, summarization, and question answering.
Why LangChain?
The growing number of models and related components for AI applications has resulted in a wide variety of different APIs that developers need to learn and use. This diversity can make it challenging for developers to switch between components or combine components when building applications.
The goal of LangChain is to streamline and facilitate the building of applications by providing key components and abstractions. It abstracts away some of the complexity of interacting with different LLMs, data sources, and external APIs while allowing for the easier creation of complex workflows.
Features
There are several primary needs that LangChain aims to address:
- Standardized component interfaces: The growing number of models and related components for AI applications has resulted in a wide variety of different APIs that developers need to learn and use. This diversity can make it challenging for developers to switch between providers or combine components when building applications. LangChain exposes a standard interface for key components, making it easy to switch between providers.
- Orchestration: As applications become more complex, combining multiple components and models, there’s a growing need to efficiently connect these elements into control flows that can accomplish diverse tasks. Orchestration is crucial for building such applications.
- Observability and Evaluation: As applications become more complex, it becomes increasingly difficult to understand what is happening within them. Furthermore, the pace of development can become rate-limited by the paradox of choice. For example, developers often wonder how to engineer their prompt or which LLM best balances accuracy, latency, and cost. Observability and evaluations can help developers monitor their applications and rapidly answer these types of questions with confidence.
Components
LangChain has many components that facilitate development, orchestration, observability, and evaluation:
- Chains – Chains allows you to create sequences of component and operations that process inputs and outputs in a specific order. These chains can involve tasks like document retrieval, question answering, summarization, or other tasks involving language models.
- Retrievers – Retrievers are components that fetch relevant data or documents based on a user’s query. This is usually done using semantic search, where the retriever finds similar data (often using embeddings). LangChain supports using external vector stores like FAISS and Pinecone for efficient search.
- LLMs (Language Models) – LangChain integrates various language models (like OpenAI’s GPT models, Hugging Face models, etc.) to handle tasks such as generating responses, summarizing text, or performing complex NLP operations.
- Document Loaders – LangChain offers functionality to load and preprocess documents (e.g., from PDFs, websites, or text files), turning them into a format that can be used by a language model or retriever. It also allows breaking down large documents for more efficient processing.
- Text Splitters – These tools break large documents into smaller, more manageable chunks. This is particularly useful when working with models that have input size limitations, ensuring that the documents can be processed without hitting those limits.
- Prompts – LangChain helps structure prompts to guide LLMs in generating responses in a controlled and desired way. Prompts can be static or dynamic and are essential for tailoring the LLM’s output to specific tasks.
- Callbacks – Callbacks allow you to track and interact with the various stages of LangChain’s operations (such as logging output, handling errors, or customizing behavior). They enable greater flexibility and control over the execution process.
- Integration with External Tools – LangChain makes it easy to integrate with external tools, such as APIs or databases. This enables complex workflows where external systems can interact with LLMs, like fetching data from a database and using it to generate answers or other outputs.
These have rational and standardized interfaces that make them easy and intuitive to work with.
Chains
But there’s more. One of the most interesting features of LangChain are chains. But I suppose that’s also why it’s reflected in the name of the framework.
Chains are sequences of components and operations that process data in a particular order. LangChain allows you to easily create chains. It also includes pre-built chains like:
- LLMChain – The most basic chain that simply passes a prompt template to an LLM.
- TransformChain – Transforms inputs using custom functions without requiring an LLM call.
- RetrievalQA – A simple chain for querying a retriever (e.g., FAISS) to retrieve relevant documents, then using an LLM to generate answers based on the context of those documents.
- ConversationalRetrievalChain – Enhances RetrievalQA with chat history, allowing follow-up questions and context preservation across multiple interactions.
- RouterChain – Routes queries to different chains based on their content or type.
- SequentialChain – Executes multiple chains in sequence, passing outputs from one to the next.
- RefineChain – Iteratively refines an answer by sequentially incorporating information from different documents.
- MapReduceChain – Processes large documents by splitting them, processing each chunk separately, and then combining the results.
- QAWithSourcesChain – Similar to RetrievalQA but specifically designed to cite sources in responses.
- AnalyzeDocumentChain – Specialized for document analysis tasks.
- SummarizationChain – Specialized for document summarization tasks.
- SQLDatabaseChain – Translates natural language to SQL queries, executes them, and returns results as natural language.
- APIChain – Interacts with external APIs based on natural language instructions.
- HypotheticalDocumentEmbedder – Generates hypothetical documents based on queries to improve embedding quality.
RetrievalQA
Let’s take a look a one particular chain, RetrievalQA. It is a specialized chain that combines the power of a retriever (such as FAISS or a document index) with a language model (LLM) to answer questions by leveraging external documents or data. It simplifies the process of building a text-based system where a model can answer questions based on information retrieved from a dataset, database, or document collection. In short, it makes it easy to make Retrieval Augmented Generation (RAG) applications.
The components of RetrievalQA are the following:
- Retriever: This is the component responsible for retrieving relevant documents or data based on the query. The retriever typically performs a semantic search to find the most relevant content for the given input. In the context of RetrievalQA, the retriever can be connected to a vector store (like FAISS or Pinecone) where documents are indexed as vectors, enabling efficient similarity searches.
- Augmentation: When a query is input, the retriever looks for the most relevant documents. These documents are then used to form the context for the LLM. The context is essentially the background information that the model uses to provide an accurate and relevant response to the query.
- LLM (Language Model): The LLM is used to generate the answer based on the context retrieved by the retriever. In RetrievalQA, the retrieved documents are fed to the LLM, which then generates a response that directly addresses the query by drawing from the provided context.
Application
In a previous post, we wrote a legal reference RAG application that allows us to ask questions about the Revised Corporation Code. The workflow is composed of several steps and we had to implement the components in the workflow:
while True:
query = input("Ask a legal question (or type 'exit' to quit): ")
if query.lower() == 'exit':
break
try:
# 1. Retrieve
relevant_info = search_faiss(model_name, query, chunks, index)
# 2. Augment
context = "\n".join(relevant_info)
# 3. Generate
response = generate_response(llm, query, context)
print("\nGemini's Answer:")
print(response)
except Exception as e:
print(f"An error occurred: {e}")
This can be simplified by using the pre-built RetrievalQA chain like so:
qa = RetrievalQA.from_chain_type(
llm=llm,
chain_type="stuff",
retriever=index.as_retriever(),
chain_type_kwargs={"prompt": PromptTemplate(input_variables=["context", "question"], template="You are a legal expert. Use the context below to answer the question clearly and concisely.\n\nContext:\n{context}\n\nQuestion:\n{question}\n\nAnswer:")}
)
# Interactive search
while True:
query = input("Ask a legal question (or type 'exit' to quit): ")
if query.lower() == 'exit':
break
try:
response = qa.invoke(query)
print("\nGemini's Answer:")
print(response["result"])
except Exception as e:
print(f"An error occurred: {e}")
To rewrite the application using LangChain, first install the packages:
pip install dotenv langchain langchain-community langchain-google-genai langchain-huggingface faiss-cpu pypdf
And here’s the full code. It is also available in the GitHub repo.
import os
from dotenv import load_dotenv
from langchain.callbacks import StreamingStdOutCallbackHandler
from langchain.chains import RetrievalQA
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.prompts import PromptTemplate
from langchain_community.document_loaders import PyPDFLoader
from langchain_community.vectorstores import FAISS
from langchain_google_genai import ChatGoogleGenerativeAI
from langchain_google_genai import GoogleGenerativeAIEmbeddings
# Load env variables
load_dotenv()
GOOGLE_API_KEY = os.getenv("GOOGLE_API_KEY")
if not GOOGLE_API_KEY:
raise ValueError("Missing API key! Set GOOGLE_API_KEY in your .env file.")
# Load the PDF and extract text
def extract_documents_from_pdf(pdf_path):
if not os.path.exists(pdf_path):
raise FileNotFoundError(f"PDF file not found: {pdf_path}")
loader = PyPDFLoader(pdf_path)
documents = loader.load()
return documents
# Split the documents into chunks
def chunk_text(documents):
text_splitter = RecursiveCharacterTextSplitter(chunk_size=2000, chunk_overlap=200)
chunks = text_splitter.split_documents(documents)
return chunks
# Generate embeddings and store in FAISS
def create_faiss_index(model, faiss_index_path, chunks):
index = FAISS.from_documents(chunks, model)
# index = FAISS.from_documents(chunks, model, index_factory="HNSW32")
index.save_local(faiss_index_path)
return index
# Main
pdf_path = "philippines-2019-legislation-ra-11232-revised-corporation-code-2019.pdf"
faiss_index_path = "faiss.index"
model_name = "models/text-embedding-004"
llm_name = 'gemini-1.5-pro-latest' # 'gemini-pro' or 'gemini-1.5-pro-latest'
# Embedding Model
embedding_model = GoogleGenerativeAIEmbeddings(model=model_name, google_api_key=GOOGLE_API_KEY)
# Load the vector store if it exists, otherwise create it
if os.path.exists(faiss_index_path):
print("Loading existing FAISS index...")
index = FAISS.load_local(faiss_index_path, embedding_model, allow_dangerous_deserialization=True)
else:
print("Creating new FAISS index...")
# Extract text, chunk, and create the FAISS index
documents = extract_documents_from_pdf(pdf_path)
chunks = chunk_text(documents)
index = create_faiss_index(embedding_model, faiss_index_path, chunks)
# LLM
llm = ChatGoogleGenerativeAI(model=llm_name, google_api_key=GOOGLE_API_KEY, temperature=0.3, callbacks=[StreamingStdOutCallbackHandler()])
qa = RetrievalQA.from_chain_type(
llm=llm,
chain_type="stuff",
retriever=index.as_retriever(),
chain_type_kwargs={"prompt": PromptTemplate(input_variables=["context", "question"], template="You are a legal expert. Use the context below to answer the question clearly and concisely.\n\nContext:\n{context}\n\nQuestion:\n{question}\n\nAnswer:")}
)
# Interactive search
while True:
query = input("Ask a legal question (or type 'exit' to quit): ")
if query.lower() == 'exit':
break
try:
response = qa.invoke(query)
print("\nGemini's Answer:")
print(response["result"])
except Exception as e:
print(f"An error occurred: {e}")
As you saw, LangChain was able to simplify our already simple application. This ease and convenience becomes even more pronounced for more complex applications.
Summary
This is just a brief introduction to LangChain. It’s a big framework and there’s a lot to be covered. But in a nutshell, LangChain simplifies LLM-based application development by providing structure and components for retrieval, orchestration, and automation. With components like Chains, Retrievers, and Prompts, it streamlines complex workflows, making AI integrations simpler and easier.