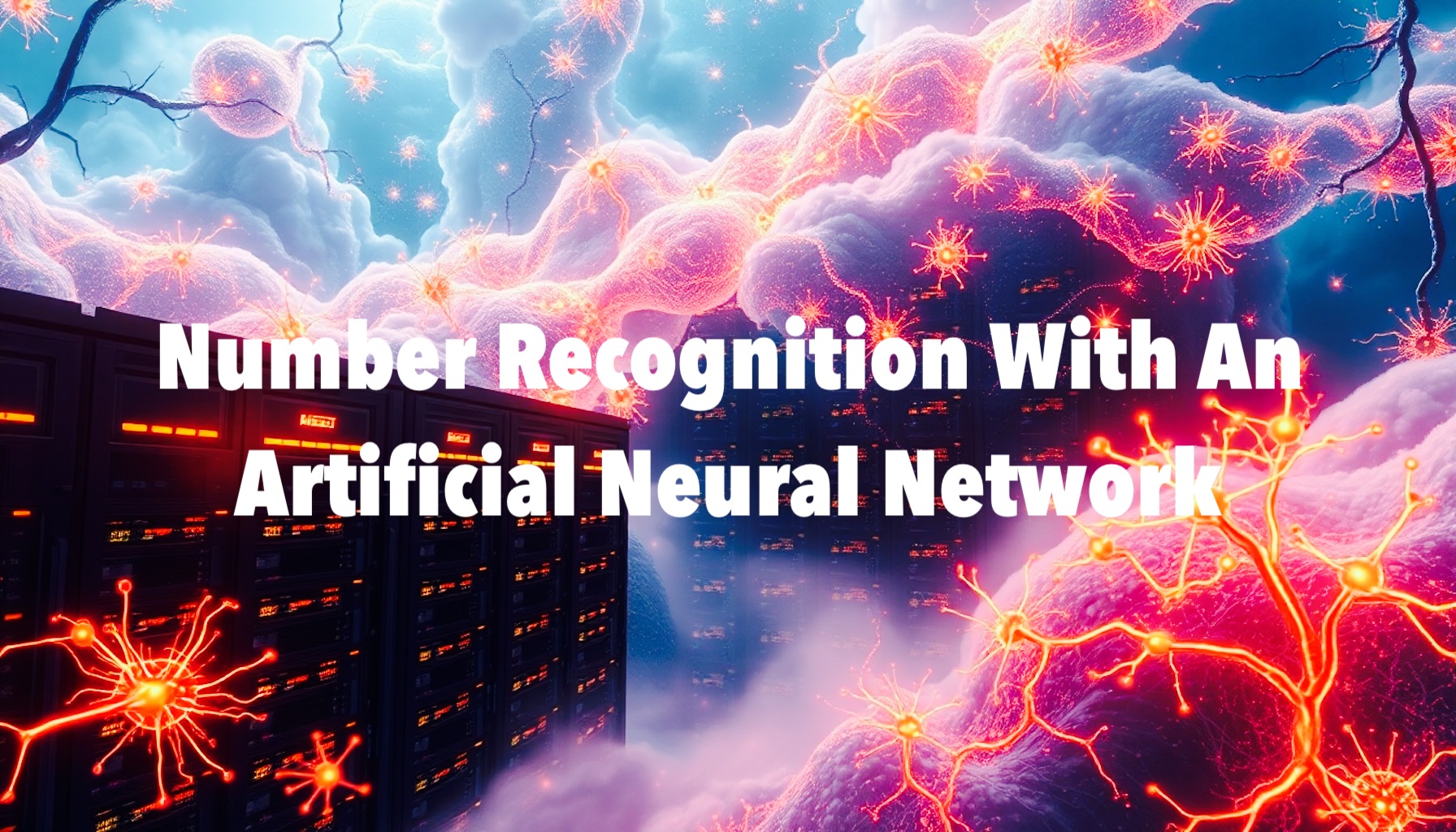
We have previously created and trained a basic artificial neural network (ANN). If you haven’t gone through that post on, you can do so now. In this post, we’ll continue and go through the process of recognizing numbers utilizing the ANN model that we created.
First, we’ll prepare some sample images that we do number recognition on. For simplicity, I extracted the sample images from the MNIST test (not training) data. The sample images as well as the script for extracting them are available on the GitHub repo.
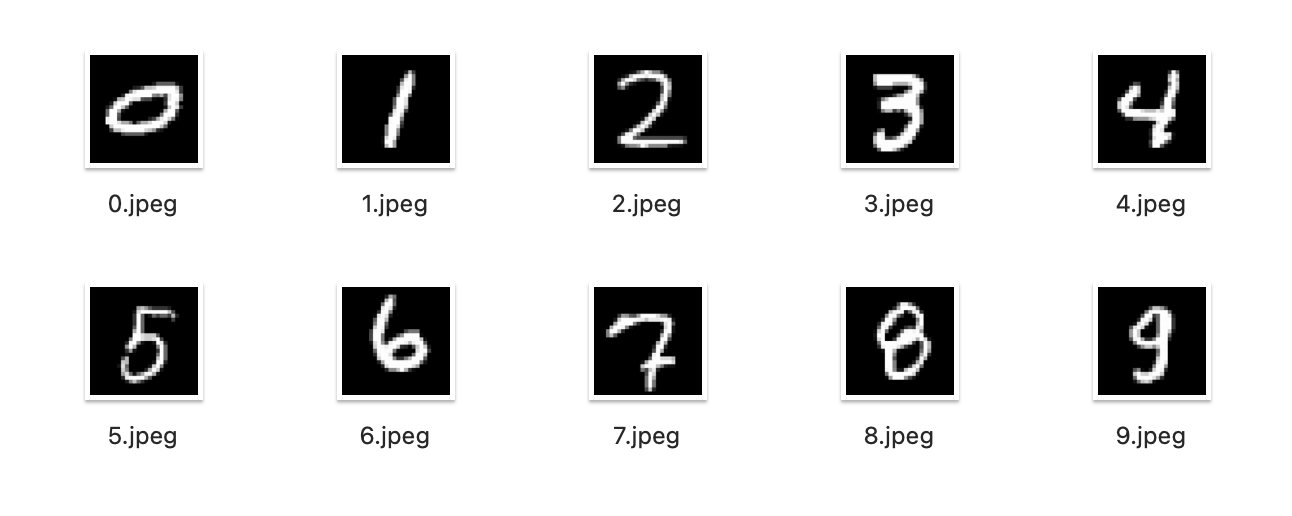
Now that we have the data, let’s install NumPy. NumPy or Numerical Python is a package for scientific computing in Python.
pip3 install numpy
Now that we have numpy, fire up your favorite editor importing the necessary libraries and modules.
import tensorflow as tf
from tensorflow.keras.preprocessing import image
import numpy as np
import argparse
Next, we load the model we created.
# Load the pre-trained model
model = tf.keras.models.load_model('model.keras')
Next , we create a recognition function to recognized the digit.
# Function to preprocess and predict the digit from images
def recognize_digit(image_path):
# Load the image
img = image.load_img(image_path, color_mode='grayscale', target_size=(28, 28))
# Convert to array and normalize
img_array = image.img_to_array(img) / 255.0
img_array = np.expand_dims(img_array, axis=0) # Add batch dimension
# Make prediction
predictions = model.predict(img_array)
predicted_class = np.argmax(predictions) # Get the index of the highest probability
return predicted_class
The function loads the image, convert it to an array, normalizes the values to 0..1, and adds a batch dimension.
The batch dimension is necessary because the Keras model’s predict() function expects the input data to be of the form (batch_size, height, width, channels). The data will be processed as a batch, even if it’s just a single image.
Next, we retrieve the filename(s) as a command line parameter and run the recognition function on each file.
if __name__ == "__main__":
# Set up argument parser
parser = argparse.ArgumentParser(description="Recognize character in 28x28 image")
parser.add_argument('input_images', nargs='+', type=str, help="Path(s) to the input color image(s) (JPG format).")
# Parse the arguments
args = parser.parse_args()
# Process each input image
for input_image in args.input_images:
print(f'Image: {input_image}')
predicted_digit = recognize_digit(input_image)
print(f'Recognized Digit: {predicted_digit}')
Finally, save the file and run it. The file is also available on the GitHub repo.
python3 recognize.py mnist_samples/*.jpeg
The results are:
Image: 0.jpeg
1/1 ???????????????????? 0s 77ms/step
Recognized Digit: 0
Image: 1.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 1
Image: 2.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 2
Image: 3.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 3
Image: 4.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 4
Image: 5.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 5
Image: 6.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 6
Image: 7.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 7
Image: 8.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 8
Image: 9.jpeg
1/1 ???????????????????? 0s 5ms/step
Recognized Digit: 9
And that’s it. We have recognized numbers with an ANN. The accuracy is excellent considering the evaluated accuracy of the model was just 89.41%!